Refund Transaction
The below code snippet must be used when implementing the Refund transaction
A refund is the process of returning funds to a customer for an already completed transaction.
It occurs when a transaction has already been approved and the payment has been taken from the customer and settled in the seller's account.
Refunds are initiated by the merchant and involve returning the funds to the customer's account.
Refunds usually happen when a customer has bought something that they’re dissatisfied with or that is no longer needed.
The Companion API allows 3rd party applications to integrate refunds of the full amount or partial amount of a previous sale transaction into their applications.
To process a refund, 3rd party developers must launch the Ecentric Payment app via an Android Intent scheme and provide the original transaction UUID in order for full / partial amounts as well as the card matching function to work correctly and then process the response intent data to understand if the refund succeeded or failed.
Note:
Should an Integrator not want the Refund matching functionality, the
originalTransactionUuid
field can simply be left blank and an Unmatched Refund transaction will be performed.However, it is highly important to enquire if your Merchant is permitted to process this particular type refund.
The below diagram shows a high-level transaction flow of a Refund transaction from the 3rd party application to the acquirer:
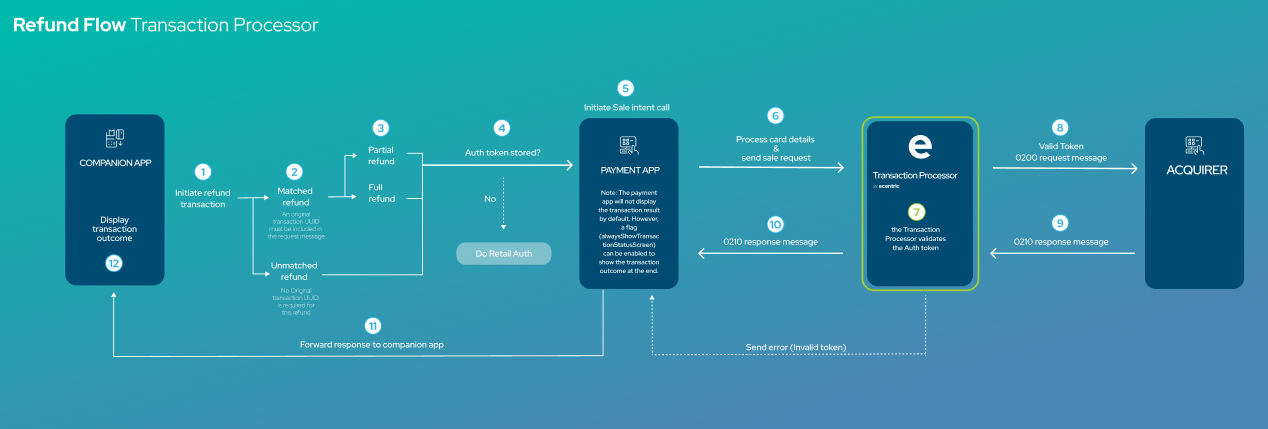
Description:
Step 1: A refund transaction is initiated on the 3rd party app
Step 2: on the 3rd party app, the merchant can either select a transaction they want to refund (Matched Refund) or process a refund which only has an amount but no original transaction (Unmatched refund)
Step 3: Once the transaction to be refunded has been selected, the Merchant must enter either the full amount of the transaction value (Full refund) or a portion of the original transaction value (Partial refund)
Step4 :
3rd party party app will then check if an Authentication token is available in the request.
- If there is an Auth token available then the transaction will continue as per normal.
- If there no Auth token available then the 3rd party app will automatically initiate a "Retail Auth - validation" request and only once the Auth token has been returned successfully will the app continue to the normal sale transaction flow.
Step 2:
The Ecentric payment app will then initiate the refund request and forward the request to Ecentric Backend.
Step 3:
The Ecentric Payment app will process the card details and forward the request to Ecentric Backend
Step 4:
The Ecentric backend will the validate the Auth token in the request.
NOTE: If the token is invalidated, the Backend will return an "invalid auth token" error back to the payment app and the transaction will be aborted.
Step 5:
The ecentric backend send a 0200 financial message to the Acquirer.
Step 6:
The Acquirer will the perform the necessary checks in order to validate and process the transaction.
Once the validation of the transaction has been performed, the Acquirer will then send a 0210 financial message to Ecentric backend which contains the transaction outcome.
Step 7:
Ecentric backend will return the transaction response to the Ecentric payment app.
Step 8:
The ecentric payment app will then forward the transaction outcome to the 3rd party app.
Step 9:
The 3rd party app will display the transaction outcome to the customer
To initiate a tokenized refund request, the original sale transaction UUID must be supplied in this call. If the original sale was successfully tokenized, the Ecentric system attempts to process the refund request using the previously tokenized card details.
The requesting POS application cannot specify which type of refund to initiate.
NOTE:
An auth token must exist before a refund can be requested.
If a refund is requested and no auth code exists, then the Ecentric Payment app will automatically initiate the authorisation process before payment can begin.
Initiating a Refund API call
It is important to note that when processing a Matched Refund:
- The "originalTransactionUuid" in the Refund request code matches the "originalTransactionUuid" of the transaction you are processing a refund on.
3rd party applications need to use the following code to invoke the Ecentric Payment app in their code in order to initiate a refund request.
private String appURL = "payment.thumbzup.com";
private String appClass = "payment.thumbzup.com.IntentActivity";
Intent intent = new Intent();
intent.setClassName(appURL, appClass);
Bundle dataBundle = new Bundle();
dataBundle.putString("launchType", "REFUND");
dataBundle.putString("applicationKey", "2fdca02f-3cbe-4e8c-82ad-86a1a16b72e7");
dataBundle.putString("merchantID", "910100000000001");
dataBundle.putString("merchantUsername", "default");
dataBundle.putString("authenticationKey", "c282cdd3-59d2-42ff-8c96-826725a27e6e");
dataBundle.putString("originalTransactionUuid", "x282cdd3-59d2-42ff-8c96-826725a27e6z");
dataBundle.putLong("transactionAmount", 10000);
intent.putExtra("thumbzupBundle", dataBundle);
startActivityForResult(intent, 0);
The table below describes the input parameters that must be provided for a refund request.
Parameter | Description |
---|---|
launchType | Must be “REFUND” Used for launching the Ecentric Payment app to process a refund. |
applicationKey | The 3rd party API key that will be issued by Ecentric specific for every 3rd party application |
authenticationKey | The authentication token that was generated by the server on a successful login to the Ecentric Payment app |
merchantUsername | The specific user name that with the appropriate role to transact via a Companion app with the Ecentric Payment app |
merchantName | The name of the merchant that requested the transaction as stored at the bank |
originalTransactionUuid | Supplying the original sale UUID will initiate a matched refund. If the original sale was tokenized, the refund will be processed via the previously tokenized card holder details. |
customerName | The name of the customer that will be making payment using the Ecentric Payment app |
The amount in cents to be refunded (long) | |
transactionDescription | Reference number for the merchant’s records |
cellNumberToSMSReceipt | 0-digit cell phone number for receipt SMS destination. Can be blank. NOTE: If isReceiptRequired is true then this is a mandatory field. |
emailAddressToSendReceipt | Valid email address for receipt email destination. Can be blank. NOTE: If isReceiptRequired is true then this is a mandatory field. |
isReceiptRequired | If set to true, at least one of the receipt parameters above needs to be set. If set to false the user will not be prompted to send a receipt after payment using the Ecentric Payment app. NOTE: According to VISA and MasterCard requirements, this must always be set to true unless the app developer is providing an alternative means to send a receipt. |
alwaysShowTransactionStatusScreen | Once the Ecentric Payment app has processed a transaction there is a status screen that shows the success/failure of processing. Set this flag to true if you would like this displayed otherwise false to hide it. Default is false. |
externalSTAN | An optional systems trace number generated by some 3rd party ERP systems |
externalTransactionGUID | An optional GUID that identifies a specific transaction generated by 3rd party ERP systems |
externalInvoiceGUID | An optional GUID that identifies a particular invoice that may appear on more than one transaction |
externalTransactionDateTime | An optional date and time the transaction was generated on the 3rd party ERP systems. Has the format of “yyyy-MM-dd'T'HH:mm:ss” |
externalTerminalId | An optional terminal identifier for device configured on the 3rd party ERP system |
latitude | An optional geolocation identifier indicating the latitude position of the device |
longitude | An optional geolocation identifier indicating the longitude position of the device |
accuracy | An optional accuracy indicator of the geolocation |
transactionUuid | Unique ID provided from 3rd party integrators. Format: UUID version 4 Note: If the field was not populated by the 3rd party integrator a system generated transaction UUID will be assign for every new transaction. |
Handling the refund response from the Ecentric Payment app
Note:
The originalTransactionUuid field will be blank if no originalTransactionUuid was in the Refund request.
This however does not mean that you as the merchant will be able to process the refund transaction if you as the Merchant are not enabled for processing Unmatched Refunds on the payment device.
When the transaction is complete, 3rd party applications need to use the following code to ecover the transaction outcome and resume the 3rd party app flow accordingly:
@Override
protected void onActivityResult(int requestCode, int resCode, Intent data) {
String result = "";
Bundle b = new Bundle(data.getBundleExtra("thumbzupApplicationResponse"));
if(resCode == Activity.RESULT_OK) {
// handle ok result
String launchType = b.getString("launchType");
String isApproved = b.getString("isApproved");
String resultCode = b.getString("resultCode");
String authenticationKey = b.getString("authenticationKey");
String applicationKey = b.getString("applicationKey");
String resultDescription = b.getString("resultDescription");
String merchantName = b.getString("merchantName");
String originalTransactionUuid = b.getString("originalTransactionUuid");
String customerName = b.getString("customerName");
Long transactionAmount = b.getLong("transactionAmount");
String transactionDescription = b.getString("transactionDescription");
String transactionUuid = b.getString("transactionUuid");
String cellNumberToSMSReceipt = b.getString("cellNumberToSMSReceipt");
String emailAddressToSendReceipt = b.getString("emailAddressToSendReceipt");
boolean isReceiptRequired = b.getBoolean("isReceiptRequired");
boolean isReceiptDataAvailable = b.getBoolean("isReceiptDataAvailable");
String externalSTAN = b.getInt("externalSTAN");
String externalRRN = b.getString("externalRRN");
String externalTransactionGUID = b.getString("externalTransactionGUID");
String externalInvoiceGUID = b.getString("externalInvoiceGUID");
String externalTransactionDateTime = b.getString("externalTransactionDateTime");
String externalTerminalId = b.getString("externalTerminalId");
String latitude = b.getString("latitude");
String longitude = b.getString("longitude");
String accuracy = b.getString("accuracy");
if (isReceiptDataAvailable) {
// this represents extra data from card processing backend
Bundle receiptBundle = b.getBundle("receiptBundle");
Iterator it = receiptBundle.keySet().iterator();
while (it.hasNext()) {
// go through extra data
Object key = it.next();
Object val = receiptBundle.get(key.toString()); }
}
}
}
The table below describes the response parameters that are available from a refund request.
Parameter | Description |
---|---|
launchType | Echo of the launchType used to launch the Ecentric Payment app. |
isApproved | Represents the processing result from a card perspective of the transaction. This will indicate whether the refund will occur or not. Valid values are: ● true – card payment will be made by the issuer ● false – card payment failed due to for example invalid PIN ● error – card payment failed due to an unknown error |
resultCode | Represents the result status of the intent call to the Ecentric Payment app (NOTE: to determine if payment will occur then please use the isApproved field as a definitive answer): ● 01 – transaction successful ● 02 – transaction declined ● 03 – transaction aborted ● 04 – error This is not a result code from the acquiring bank and should not be made available to the customer at any time. For the Issuer/Acquirer response code, see field RC in the receiptBundle (see 3.6 Additional Server Fields). |
resultDescription | A user readable representation of the above resultCode i.e. Approved for 01 resultCode If the acquiring bank approves or declines the transaction, the response description is included in this field. |
merchantName | The name of the merchant that requested the transaction as stored at the bank |
originalTransactionUuid | Echo of the originalTransactionUuid used to launch the Ecentric Payment app |
customerName | Echo of the customerName used to launch the Ecentric Payment app |
applicationKey | The 3rd party API key that will be issued by Ecentric specific for every 3rd party application |
authenticationKey | The authentication token that was generated by the server on a successful login to the Ecentric Payment app |
transactionAmount | Echo of the transactionAmount used to launch the Ecentric Payment app. |
transactionDescription | Echo of the transactionDescription used to launch the Ecentric Payment app |
transactionUuid | Unique ID provided from 3rd party integrators. Format: UUID version 4 Note: If the field was not populated by the 3rd party integrator a system generated transaction UUID will be assign for every new transaction. |
cellNumberToSMSReceipt | Masked out value of the cell number used to send the receipt to i.e. ***657 |
emailAddressToSendReceipt | Masked out value of the email address used to send the receipt to i.e. **@Ecentric.com |
isReceiptRequired | Echo of the isReceiptRequired used to launch the Ecentric Payment app |
isReceiptDataAvailable | A boolean indicating whether a receiptBundle parameter is available. Will always be there for accepted or declined transactions |
receiptBundle | Consists of a sub-bundle of server parameters that can be used by the partner application. For a full list of these parameters see the Additional Server Fields section |
pebbleFirmwareVersion | The software version currently running on the Payment Pebble® |
pebbleSerialNumber | The serial number of the Payment Pebble® |
appVersion | The software version currently running on the Ecentric Payment app |
deviceIMEI | The IMEI number of the Payment Blade |
externalSTAN | Echo of the systems trace number generated by some 3rd party ERP systems |
externalRRN | Echo of the RRN generated by some 3rd party ERP systems |
externalTransactionGUID | Echo of the GUID that identifies a specific transaction generated by 3rd party ERP systems |
externalInvoiceGUID | Echo of the GUID that identifies a particular invoice that may appear on more than one transaction |
externalTransactionDateTime | Echo of the date and time the transaction was generated on the 3rd party ERP systems. Has the format of “yyyy-MM-dd'T'HH:mm:ss” |
externalTerminalId | Echo of the terminal identifier for device configured on the 3rd party ERP system |
terminalId | This is an automatically system-assigned terminalID of the payment terminal’s identity number, which can be used to assist with settlement information and is returned in BASE36 format |
latitude | Echo of geolocation identifier indicating the latitude position of the device |
longitude | Echo of geolocation identifier indicating the longitude position of the device |
accuracy | Echo of accuracy indicator of the geolocation |
Updated about 2 months ago